Class WaterfallBarRenderer
java.lang.Object
org.jfree.chart.renderer.AbstractRenderer
org.jfree.chart.renderer.category.AbstractCategoryItemRenderer
org.jfree.chart.renderer.category.BarRenderer
org.jfree.chart.renderer.category.WaterfallBarRenderer
- All Implemented Interfaces:
Serializable
,Cloneable
,LegendItemSource
,CategoryItemRenderer
,PublicCloneable
A renderer that handles the drawing of waterfall bar charts, for use with
the
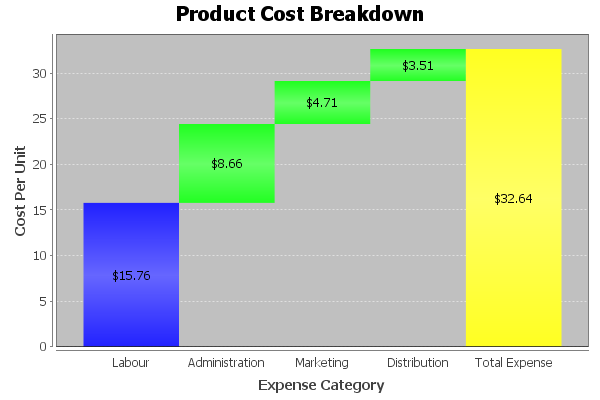
CategoryPlot
class. Some quirks to note:
- the value in the last category of the dataset should be (redundantly) specified as the sum of the items in the preceding categories - otherwise the final bar in the plot will be incorrectly plotted;
- the bar colors are defined using special methods in this class - the
inherited methods (for example,
AbstractRenderer.setSeriesPaint(int, Paint)
) are ignored;
WaterfallChartDemo1.java
program included in the JFreeChart
Demo Collection:
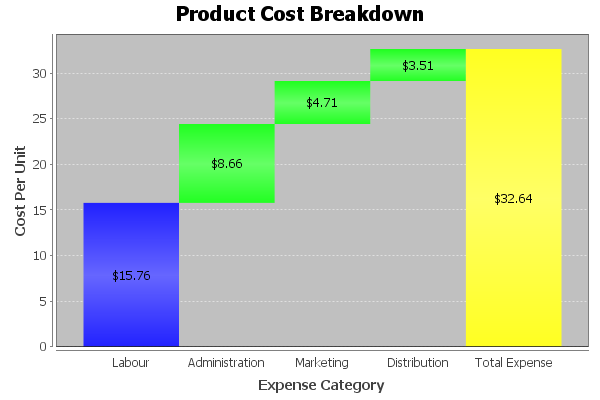
- See Also:
-
Field Summary
Fields inherited from class org.jfree.chart.renderer.category.BarRenderer
BAR_OUTLINE_WIDTH_THRESHOLD, DEFAULT_ITEM_MARGIN
Fields inherited from class org.jfree.chart.renderer.AbstractRenderer
DEFAULT_ITEM_LABEL_INSETS, DEFAULT_OUTLINE_PAINT, DEFAULT_OUTLINE_STROKE, DEFAULT_PAINT, DEFAULT_SHAPE, DEFAULT_STROKE, DEFAULT_VALUE_LABEL_FONT, DEFAULT_VALUE_LABEL_PAINT, ZERO
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs a new renderer with default values for the bar colors.WaterfallBarRenderer
(Paint firstBarPaint, Paint positiveBarPaint, Paint negativeBarPaint, Paint lastBarPaint) Constructs a new waterfall renderer. -
Method Summary
Modifier and TypeMethodDescriptionvoid
drawItem
(Graphics2D g2, CategoryItemRendererState state, Rectangle2D dataArea, CategoryPlot plot, CategoryAxis domainAxis, ValueAxis rangeAxis, CategoryDataset dataset, int row, int column, int pass) Draws the bar for a single (series, category) data item.boolean
Tests an object for equality with this instance.findRangeBounds
(CategoryDataset dataset) Returns the range of values the renderer requires to display all the items from the specified dataset.Returns the paint used to draw the first bar.Returns the paint used to draw the last bar.Returns the paint used to draw bars with negative values.Returns the paint used to draw bars with positive values.void
setFirstBarPaint
(Paint paint) Sets the paint that will be used to draw the first bar and sends aRendererChangeEvent
to all registered listeners.void
setLastBarPaint
(Paint paint) Sets the paint that will be used to draw the last bar and sends aRendererChangeEvent
to all registered listeners.void
setNegativeBarPaint
(Paint paint) Sets the paint that will be used to draw bars having negative values, and sends aRendererChangeEvent
to all registered listeners.void
setPositiveBarPaint
(Paint paint) Sets the paint that will be used to draw bars having positive values.Methods inherited from class org.jfree.chart.renderer.category.BarRenderer
calculateBarL0L1, calculateBarW0, calculateBarWidth, calculateSeriesWidth, drawItemLabel, findRangeBounds, getBarPainter, getBase, getDefaultBarPainter, getDefaultShadowsVisible, getGradientPaintTransformer, getIncludeBaseInRange, getItemMargin, getLegendItem, getLowerClip, getMaximumBarWidth, getMinimumBarLength, getNegativeItemLabelPositionFallback, getPositiveItemLabelPositionFallback, getShadowPaint, getShadowsVisible, getShadowXOffset, getShadowYOffset, getUpperClip, initialise, isDrawBarOutline, setBarPainter, setBase, setDefaultBarPainter, setDefaultShadowsVisible, setDrawBarOutline, setGradientPaintTransformer, setIncludeBaseInRange, setItemMargin, setMaximumBarWidth, setMinimumBarLength, setNegativeItemLabelPositionFallback, setPositiveItemLabelPositionFallback, setShadowPaint, setShadowVisible, setShadowXOffset, setShadowYOffset
Methods inherited from class org.jfree.chart.renderer.category.AbstractCategoryItemRenderer
addEntity, addItemEntity, beginElementGroup, calculateDomainMarkerTextAnchorPoint, calculateRangeMarkerTextAnchorPoint, clone, createState, drawBackground, drawDomainGridline, drawDomainMarker, drawItemLabel, drawOutline, drawRangeLine, drawRangeMarker, getColumnCount, getDefaultItemLabelGenerator, getDefaultItemURLGenerator, getDefaultToolTipGenerator, getDomainAxis, getDrawingSupplier, getItemLabelGenerator, getItemMiddle, getItemURLGenerator, getLegendItemLabelGenerator, getLegendItems, getLegendItemToolTipGenerator, getLegendItemURLGenerator, getPassCount, getPlot, getRangeAxis, getRowCount, getSeriesItemLabelGenerator, getSeriesItemURLGenerator, getSeriesToolTipGenerator, getToolTipGenerator, hashCode, setDefaultItemLabelGenerator, setDefaultItemLabelGenerator, setDefaultItemURLGenerator, setDefaultItemURLGenerator, setDefaultToolTipGenerator, setDefaultToolTipGenerator, setLegendItemLabelGenerator, setLegendItemToolTipGenerator, setLegendItemURLGenerator, setPlot, setSeriesItemLabelGenerator, setSeriesItemLabelGenerator, setSeriesItemURLGenerator, setSeriesItemURLGenerator, setSeriesToolTipGenerator, setSeriesToolTipGenerator, updateCrosshairValues
Methods inherited from class org.jfree.chart.renderer.AbstractRenderer
addChangeListener, beginElementGroup, calculateLabelAnchorPoint, clearSeriesPaints, clearSeriesStrokes, endElementGroup, fireChangeEvent, getAutoPopulateSeriesFillPaint, getAutoPopulateSeriesOutlinePaint, getAutoPopulateSeriesOutlineStroke, getAutoPopulateSeriesPaint, getAutoPopulateSeriesShape, getAutoPopulateSeriesStroke, getDataBoundsIncludesVisibleSeriesOnly, getDefaultCreateEntities, getDefaultEntityRadius, getDefaultFillPaint, getDefaultItemLabelFont, getDefaultItemLabelPaint, getDefaultItemLabelsVisible, getDefaultLegendShape, getDefaultLegendTextFont, getDefaultLegendTextPaint, getDefaultNegativeItemLabelPosition, getDefaultOutlinePaint, getDefaultOutlineStroke, getDefaultPaint, getDefaultPositiveItemLabelPosition, getDefaultSeriesVisible, getDefaultSeriesVisibleInLegend, getDefaultShape, getDefaultStroke, getItemCreateEntity, getItemFillPaint, getItemLabelAnchorOffset, getItemLabelFont, getItemLabelInsets, getItemLabelPaint, getItemOutlinePaint, getItemOutlineStroke, getItemPaint, getItemShape, getItemStroke, getItemVisible, getLegendShape, getLegendTextFont, getLegendTextPaint, getNegativeItemLabelPosition, getPositiveItemLabelPosition, getSeriesCreateEntities, getSeriesFillPaint, getSeriesItemLabelFont, getSeriesItemLabelPaint, getSeriesNegativeItemLabelPosition, getSeriesOutlinePaint, getSeriesOutlineStroke, getSeriesPaint, getSeriesPositiveItemLabelPosition, getSeriesShape, getSeriesStroke, getSeriesVisible, getSeriesVisibleInLegend, getTreatLegendShapeAsLine, hasListener, isComputeItemLabelContrastColor, isItemLabelVisible, isSeriesItemLabelsVisible, isSeriesVisible, isSeriesVisibleInLegend, lookupLegendShape, lookupLegendTextFont, lookupLegendTextPaint, lookupSeriesFillPaint, lookupSeriesOutlinePaint, lookupSeriesOutlineStroke, lookupSeriesPaint, lookupSeriesShape, lookupSeriesStroke, notifyListeners, removeChangeListener, setAutoPopulateSeriesFillPaint, setAutoPopulateSeriesOutlinePaint, setAutoPopulateSeriesOutlineStroke, setAutoPopulateSeriesPaint, setAutoPopulateSeriesShape, setAutoPopulateSeriesStroke, setComputeItemLabelContrastColor, setDataBoundsIncludesVisibleSeriesOnly, setDefaultCreateEntities, setDefaultCreateEntities, setDefaultEntityRadius, setDefaultFillPaint, setDefaultFillPaint, setDefaultItemLabelFont, setDefaultItemLabelFont, setDefaultItemLabelPaint, setDefaultItemLabelPaint, setDefaultItemLabelsVisible, setDefaultItemLabelsVisible, setDefaultLegendShape, setDefaultLegendTextFont, setDefaultLegendTextPaint, setDefaultNegativeItemLabelPosition, setDefaultNegativeItemLabelPosition, setDefaultOutlinePaint, setDefaultOutlinePaint, setDefaultOutlineStroke, setDefaultOutlineStroke, setDefaultPaint, setDefaultPaint, setDefaultPositiveItemLabelPosition, setDefaultPositiveItemLabelPosition, setDefaultSeriesVisible, setDefaultSeriesVisible, setDefaultSeriesVisibleInLegend, setDefaultSeriesVisibleInLegend, setDefaultShape, setDefaultShape, setDefaultStroke, setDefaultStroke, setItemLabelAnchorOffset, setItemLabelInsets, setLegendShape, setLegendTextFont, setLegendTextPaint, setSeriesCreateEntities, setSeriesCreateEntities, setSeriesFillPaint, setSeriesFillPaint, setSeriesItemLabelFont, setSeriesItemLabelFont, setSeriesItemLabelPaint, setSeriesItemLabelPaint, setSeriesItemLabelsVisible, setSeriesItemLabelsVisible, setSeriesItemLabelsVisible, setSeriesNegativeItemLabelPosition, setSeriesNegativeItemLabelPosition, setSeriesOutlinePaint, setSeriesOutlinePaint, setSeriesOutlineStroke, setSeriesOutlineStroke, setSeriesPaint, setSeriesPaint, setSeriesPositiveItemLabelPosition, setSeriesPositiveItemLabelPosition, setSeriesShape, setSeriesShape, setSeriesStroke, setSeriesStroke, setSeriesVisible, setSeriesVisible, setSeriesVisibleInLegend, setSeriesVisibleInLegend, setTreatLegendShapeAsLine
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.jfree.chart.renderer.category.CategoryItemRenderer
addChangeListener, getDefaultCreateEntities, getDefaultFillPaint, getDefaultItemLabelFont, getDefaultItemLabelPaint, getDefaultItemLabelsVisible, getDefaultNegativeItemLabelPosition, getDefaultOutlinePaint, getDefaultOutlineStroke, getDefaultPaint, getDefaultPositiveItemLabelPosition, getDefaultSeriesVisible, getDefaultSeriesVisibleInLegend, getDefaultShape, getDefaultStroke, getItemCreateEntity, getItemFillPaint, getItemLabelFont, getItemLabelPaint, getItemOutlinePaint, getItemOutlineStroke, getItemPaint, getItemShape, getItemStroke, getItemVisible, getNegativeItemLabelPosition, getPositiveItemLabelPosition, getSeriesCreateEntities, getSeriesFillPaint, getSeriesItemLabelFont, getSeriesItemLabelPaint, getSeriesNegativeItemLabelPosition, getSeriesOutlinePaint, getSeriesOutlineStroke, getSeriesPaint, getSeriesPositiveItemLabelPosition, getSeriesShape, getSeriesStroke, getSeriesVisible, getSeriesVisibleInLegend, isItemLabelVisible, isSeriesItemLabelsVisible, isSeriesVisible, isSeriesVisibleInLegend, removeChangeListener, setDefaultCreateEntities, setDefaultCreateEntities, setDefaultFillPaint, setDefaultItemLabelFont, setDefaultItemLabelFont, setDefaultItemLabelPaint, setDefaultItemLabelPaint, setDefaultItemLabelsVisible, setDefaultItemLabelsVisible, setDefaultNegativeItemLabelPosition, setDefaultNegativeItemLabelPosition, setDefaultOutlinePaint, setDefaultOutlinePaint, setDefaultOutlineStroke, setDefaultOutlineStroke, setDefaultPaint, setDefaultPaint, setDefaultPositiveItemLabelPosition, setDefaultPositiveItemLabelPosition, setDefaultSeriesVisible, setDefaultSeriesVisible, setDefaultSeriesVisibleInLegend, setDefaultSeriesVisibleInLegend, setDefaultShape, setDefaultShape, setDefaultStroke, setDefaultStroke, setSeriesCreateEntities, setSeriesCreateEntities, setSeriesFillPaint, setSeriesItemLabelFont, setSeriesItemLabelFont, setSeriesItemLabelPaint, setSeriesItemLabelPaint, setSeriesItemLabelsVisible, setSeriesItemLabelsVisible, setSeriesItemLabelsVisible, setSeriesNegativeItemLabelPosition, setSeriesNegativeItemLabelPosition, setSeriesOutlinePaint, setSeriesOutlinePaint, setSeriesOutlineStroke, setSeriesOutlineStroke, setSeriesPaint, setSeriesPaint, setSeriesPositiveItemLabelPosition, setSeriesPositiveItemLabelPosition, setSeriesShape, setSeriesShape, setSeriesStroke, setSeriesStroke, setSeriesVisible, setSeriesVisible, setSeriesVisibleInLegend, setSeriesVisibleInLegend
Methods inherited from interface org.jfree.chart.util.PublicCloneable
clone
-
Constructor Details
-
WaterfallBarRenderer
public WaterfallBarRenderer()Constructs a new renderer with default values for the bar colors. -
WaterfallBarRenderer
public WaterfallBarRenderer(Paint firstBarPaint, Paint positiveBarPaint, Paint negativeBarPaint, Paint lastBarPaint) Constructs a new waterfall renderer.- Parameters:
firstBarPaint
- the color of the first bar (null
not permitted).positiveBarPaint
- the color for bars with positive values (null
not permitted).negativeBarPaint
- the color for bars with negative values (null
not permitted).lastBarPaint
- the color of the last bar (null
not permitted).
-
-
Method Details
-
getFirstBarPaint
Returns the paint used to draw the first bar.- Returns:
- The paint (never
null
).
-
setFirstBarPaint
Sets the paint that will be used to draw the first bar and sends aRendererChangeEvent
to all registered listeners.- Parameters:
paint
- the paint (null
not permitted).
-
getLastBarPaint
Returns the paint used to draw the last bar.- Returns:
- The paint (never
null
).
-
setLastBarPaint
Sets the paint that will be used to draw the last bar and sends aRendererChangeEvent
to all registered listeners.- Parameters:
paint
- the paint (null
not permitted).
-
getPositiveBarPaint
Returns the paint used to draw bars with positive values.- Returns:
- The paint (never
null
).
-
setPositiveBarPaint
Sets the paint that will be used to draw bars having positive values.- Parameters:
paint
- the paint (null
not permitted).
-
getNegativeBarPaint
Returns the paint used to draw bars with negative values.- Returns:
- The paint (never
null
).
-
setNegativeBarPaint
Sets the paint that will be used to draw bars having negative values, and sends aRendererChangeEvent
to all registered listeners.- Parameters:
paint
- the paint (null
not permitted).
-
findRangeBounds
Returns the range of values the renderer requires to display all the items from the specified dataset.- Specified by:
findRangeBounds
in interfaceCategoryItemRenderer
- Overrides:
findRangeBounds
in classAbstractCategoryItemRenderer
- Parameters:
dataset
- the dataset (null
not permitted).- Returns:
- The range (or
null
if the dataset is empty).
-
drawItem
public void drawItem(Graphics2D g2, CategoryItemRendererState state, Rectangle2D dataArea, CategoryPlot plot, CategoryAxis domainAxis, ValueAxis rangeAxis, CategoryDataset dataset, int row, int column, int pass) Draws the bar for a single (series, category) data item.- Specified by:
drawItem
in interfaceCategoryItemRenderer
- Overrides:
drawItem
in classBarRenderer
- Parameters:
g2
- the graphics device.state
- the renderer state.dataArea
- the data area.plot
- the plot.domainAxis
- the domain axis.rangeAxis
- the range axis.dataset
- the dataset.row
- the row index (zero-based).column
- the column index (zero-based).pass
- the pass index.
-
equals
Tests an object for equality with this instance.- Overrides:
equals
in classBarRenderer
- Parameters:
obj
- the object (null
permitted).- Returns:
- A boolean.
-